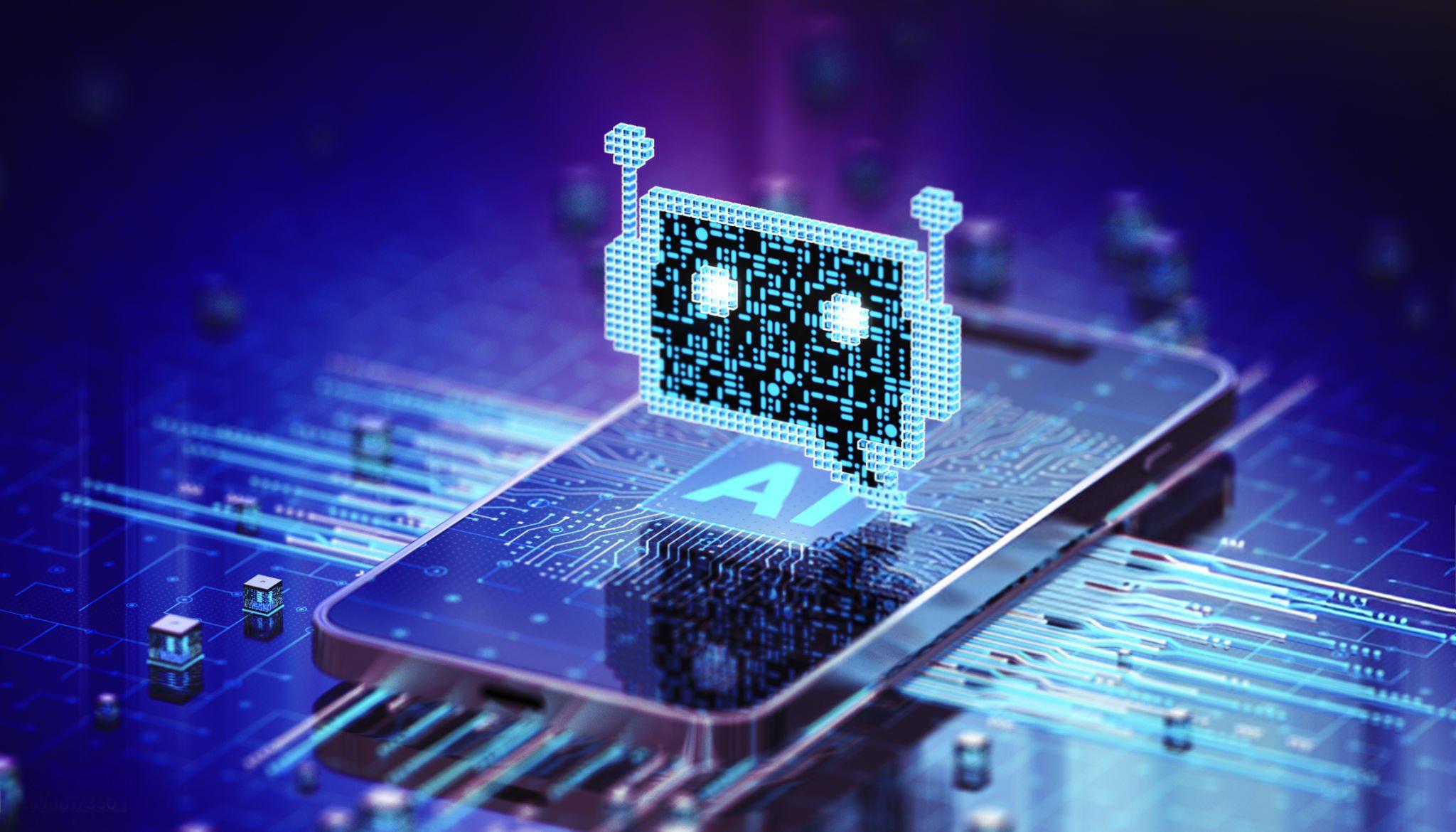
Chatbots have become increasingly popular in recent years, thanks to advancements in natural language processing and machine learning. With the OpenAI API, building a chatbot has never been easier.
In this blog, we’ll take a closer look at a Python script that uses the OpenAI API to create a chatbot that can respond to user input. The chatbot will start by asking the user how they feel, and will then provide responses based on the user’s input.
To get started, we need to import the openai
module and set the API key.
import openai
openai.api_key = "[OPENAI_API_KEY]"
Next, we define a list of messages that will be used to store the conversation history. The first message in the list is a system message that describes the chatbot’s role.
messages = [
{
"role": "system",
"content": "[CAHTBOT ROLE DESCRIPTION]"
}
]
After defining the messages, we print a prompt for the user to input their feelings.
print("how do you feel?")
We then enter into a while
loop that will continue until the user decides to exit the conversation. Within the loop, we ask the user to input their message, add it to the list of messages, and send the message to the OpenAI API for processing.
while True:
msg = input()
messages.append(
{
"role": "user",
"content": msg,
}
)
resp = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=messages
)
The response from the OpenAI API is then added to the list of messages and printed to the user.
ans = resp.choices[0].message.content
messages.append(
{
"role": "assistant",
"content": ans,
}
)
print(ans)
Here’s the full code:
import openai
openai.api_key = "[OPENAI_API_KEY]"
messages = [
{
"role": "system",
"content": "[CAHTBOT ROLE DESCRIPTION]"
}
]
print("how do you feel?")
while True:
msg = input()
messages.append(
{
"role": "user",
"content": msg,
}
)
resp = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=messages
)
ans = resp.choices[0].message.content
messages.append(
{
"role": "assistant",
"content": ans,
}
)
print(ans)
By using the OpenAI API and Python, we can easily create chatbots that can provide personalized responses to users. With a few lines of code, we can simulate a conversation with the user and provide them with the assistance they need.
Live Demo
In conclusion, this Python script shows how easy it is to create a chatbot using the OpenAI API. With natural language processing and machine learning, chatbots are becoming more advanced, and can provide valuable assistance to users.